Roman Numeral Converter
Convert the given number into a roman numeral.
All roman numerals answers should be provided in upper-case.
SOLUTION:
let romanNumbers = { 1: "I", 4: "IV", 5: "V", 9: "IX", 10: "X", 40: "XL", 50: "L", 90: "XC", 100: "C", 400: "CD", 500: "D", 900: "CM", 1000: "M" } let diffRoman = { 10: 1, 100: 10, 1000: 100 } function convertToRoman(num) { let ch = factorNum(num).map(item => { let i = romanNumbers[item] return i }) return ch.join(""); }
function factorNum(num, arr = []) { let numFactors = [...arr] let a = Object.keys(romanNumbers).sort((a,b) => b-a).filter(item => num>=item)[0] numFactors.push(a) let b = num-a; let c = Object.keys(diffRoman).sort((a,b) => a-b).filter(item => b < item)[0] let d = diffRoman[c]; let e = Object.keys(romanNumbers).sort((a,b) => b-a) if(e.includes(b+d)) { numFactors.push(b.toString()) } else if(b > 1) { return factorNum(b, numFactors) } else { numFactors.push(b.toString()) } return numFactors; }
// convertToRoman(36); console.log(convertToRoman(68)) |
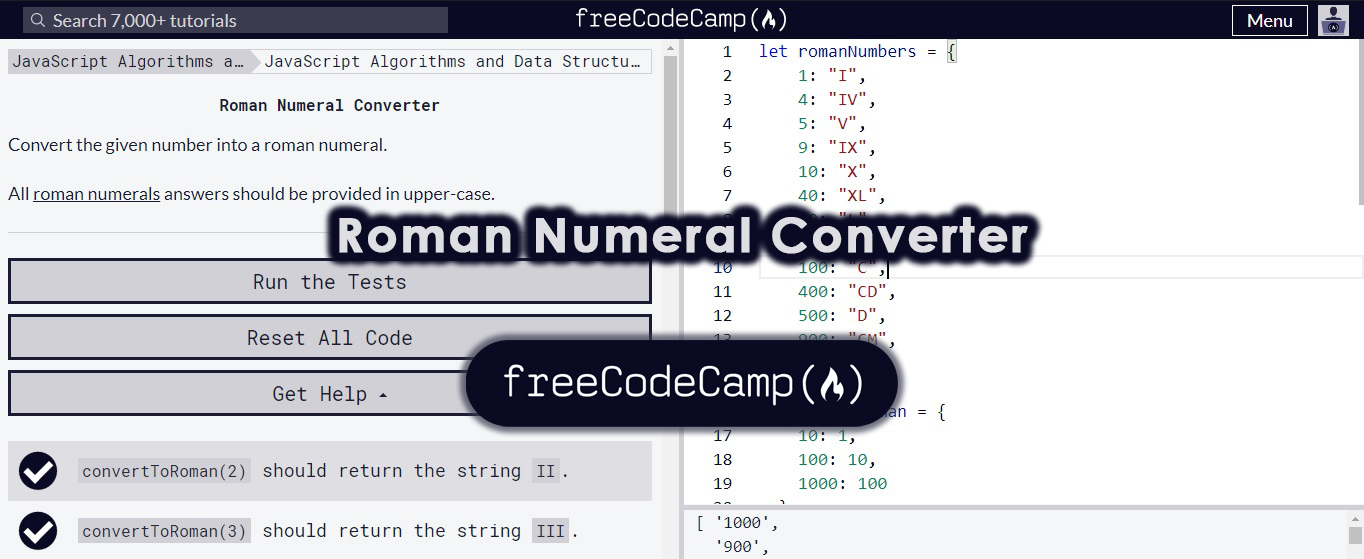
Click here to go to the original link of the question.